Add Events in the Allowances Smart Contract¶
One thing that's missing is events.
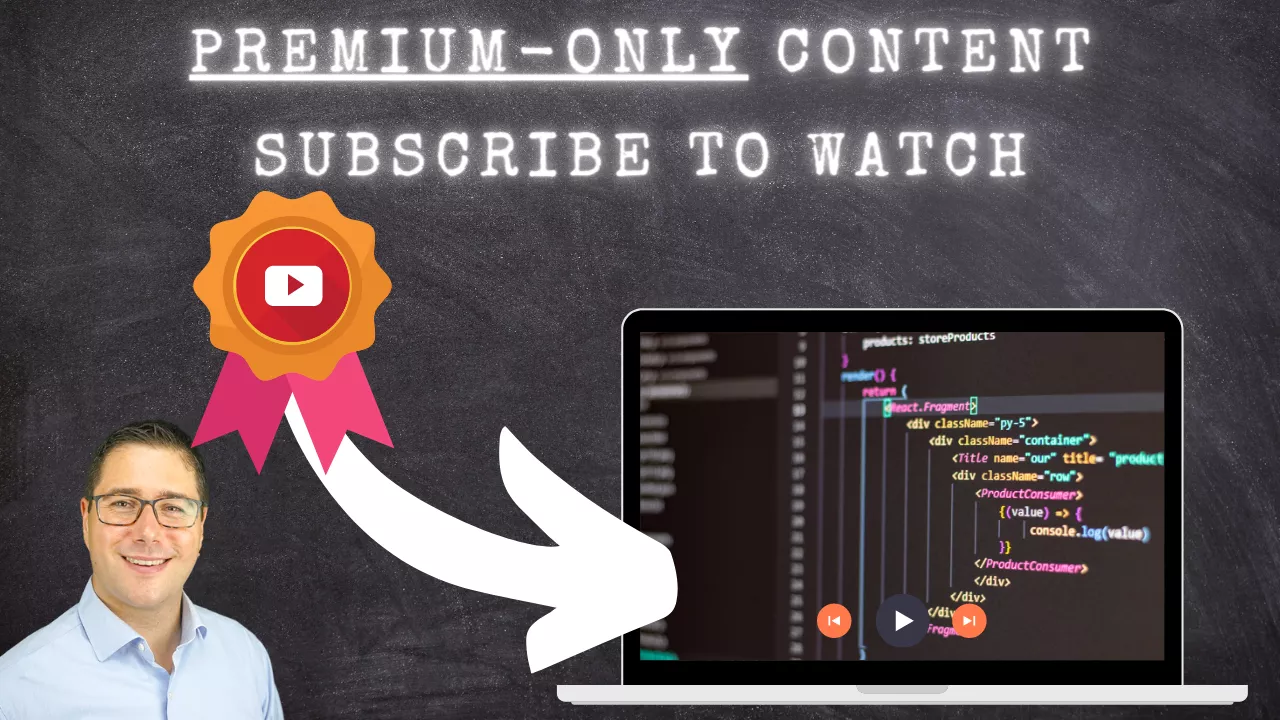
//SPDX-License-Identifier: MIT
pragma solidity 0.8.1;
import "https://github.com/OpenZeppelin/openzeppelin-contracts/blob/master/contracts/access/Ownable.sol";
contract Allowance is Ownable {
event AllowanceChanged(address indexed _forWho, address indexed _byWhom, uint _oldAmount, uint _newAmount);
mapping(address => uint) public allowance;
function isOwner() internal view returns(bool) {
return owner() == msg.sender;
}
function setAllowance(address _who, uint _amount) public onlyOwner {
emit AllowanceChanged(_who, msg.sender, allowance[_who], _amount);
allowance[_who] = _amount;
}
modifier ownerOrAllowed(uint _amount) {
require(isOwner() || allowance[msg.sender] >= _amount, "You are not allowed!");
_;
}
function reduceAllowance(address _who, uint _amount) internal ownerOrAllowed(_amount) {
emit AllowanceChanged(_who, msg.sender, allowance[_who], allowance[_who] - _amount);
allowance[_who] -= _amount;
}
}
contract SharedWallet is Allowance {
//…
}
Last update:
April 29, 2022