Ownable Functionality¶
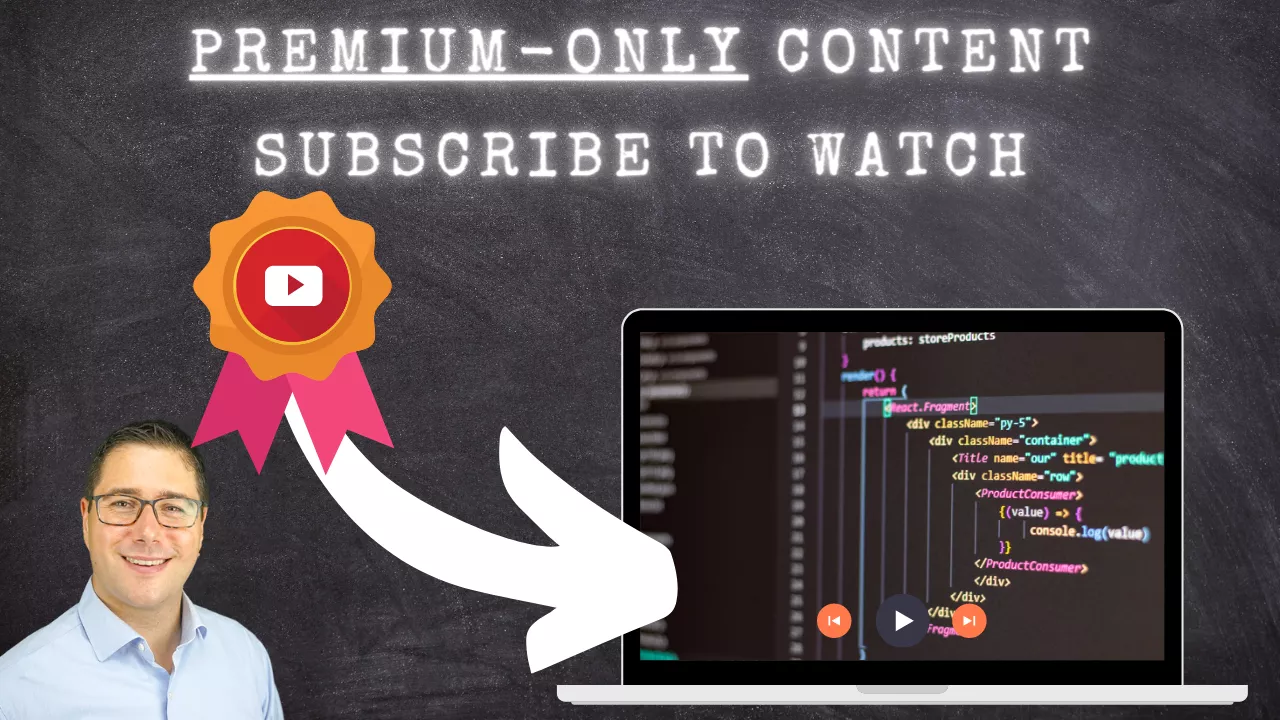
Normally we would add the OpenZeppelin Smart Contracts with the Ownable Functionality. But at the time of writing this document they are not updated to solidity 0.6 yet. So, instead we will add our own Ownable functionality very much like the one from OpenZeppelin:
pragma solidity ^0.6.0;
contract Ownable {
address public _owner;
constructor () internal {
_owner = msg.sender;
}
/**
* @dev Throws if called by any account other than the owner.
*/
modifier onlyOwner() {
require(isOwner(), "Ownable: caller is not the owner");
_;
}
/**
* @dev Returns true if the caller is the current owner.
*/
function isOwner() public view returns (bool) {
return (msg.sender == _owner);
}
}
Then modify the ItemManager so that all functions, that should be executable by the "owner only" have the correct modifier:
pragma solidity ^0.6.0;
import "./Ownable.sol";
import "./Item.sol";
contract ItemManager is Ownable {
//…
function createItem(string memory _identifier, uint _priceInWei) public onlyOwner {
//…
}
function triggerPayment(uint _index) public payable {
//…
}
function triggerDelivery(uint _index) public onlyOwner {
//…
}
Last update:
April 29, 2022