Change the UnitTests To Support TokenSales¶
The truffle tests setup is not really suitable if you want to test specific scenarios which are not covered by the migration files. After migrating a smart contract, it usually ends up in a specific state. So testing the Token Smart Contract in this way wouldn't be possible anymore. You would have to test the whole token-sale, but that's something not what we want.
We could also integrate the openzeppelin test environment. It's blazing fast and comes with an internal blockchain for testing. But it has one large drawback: It only let's you use the internal blockchain, it's not configurable so it would use an outside blockchain. That's why I would still opt to use the Truffle Environment.
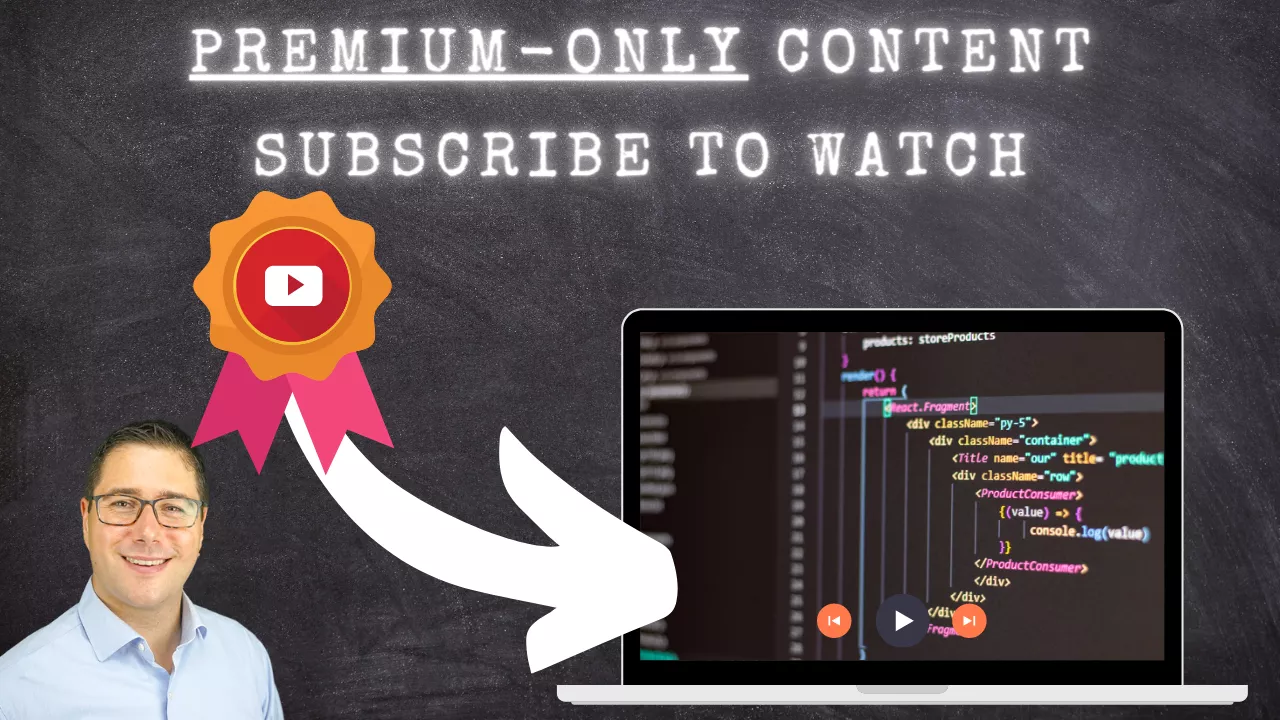
We just have to make a small change:
//… chai token setup
contract("Token Test", function(accounts) {
const [ initialHolder, recipient, anotherAccount ] = accounts;
beforeEach(async () => {
this.myToken = await Token.new(1000);
});
it("All tokens should be in my account", async () => {
//let instance = await Token.deployed();
let instance = this.myToken;
let totalSupply = await instance.totalSupply();
//… more content
});
it("I can send tokens from Account 1 to Account 2", async () => {
const sendTokens = 1;
let instance = this.myToken;
let totalSupply = await instance.totalSupply();
//… more content
});
it("It's not possible to send more tokens than account 1 has", async () => {
let instance = this.myToken;
//… more content
});
});
Now open your Terminal and test the smart contract:
Add in a Central Configuration with DotEnv¶
One of the larger problems is that we now have a constant for the migrations-file and a constant in our test -- the amount of tokens that are created. It would be better to have this constant through an environment file.
Install Dot-Env:
npm install --save dotenv
Then create a new file .env in your root directory of the project with the following content:
INITIAL_TOKENS = 10000000
Then change the migrations file to:
var MyToken = artifacts.require("./MyToken.sol");
var MyTokenSales = artifacts.require("./MyTokenSale.sol");
require('dotenv').config({path: '../.env'});
module.exports = async function(deployer) {
let addr = await web3.eth.getAccounts();
await deployer.deploy(MyToken, process.env.INITIAL_TOKENS);
await deployer.deploy(MyTokenSales, 1, addr[0], MyToken.address);
let tokenInstance = await MyToken.deployed();
await tokenInstance.transfer(MyTokenSales.address, process.env.INITIAL_TOKENS);
};
Update also the tests file:
const Token = artifacts.require("MyToken");
// Rest of the code ...
require('dotenv').config({path: '../.env'});
contract("Token Test", function(accounts) {
const [ initialHolder, recipient, anotherAccount ] = accounts;
beforeEach(async () => {
this.myToken = await Token.new(process.env.INITIAL_TOKENS);
});
//Rest of the Code ...
Now run the tests again and make sure everything still works as expected! All the tests, as well as the migration itself have one single point of truth. That is the .env file.