Update KYC¶
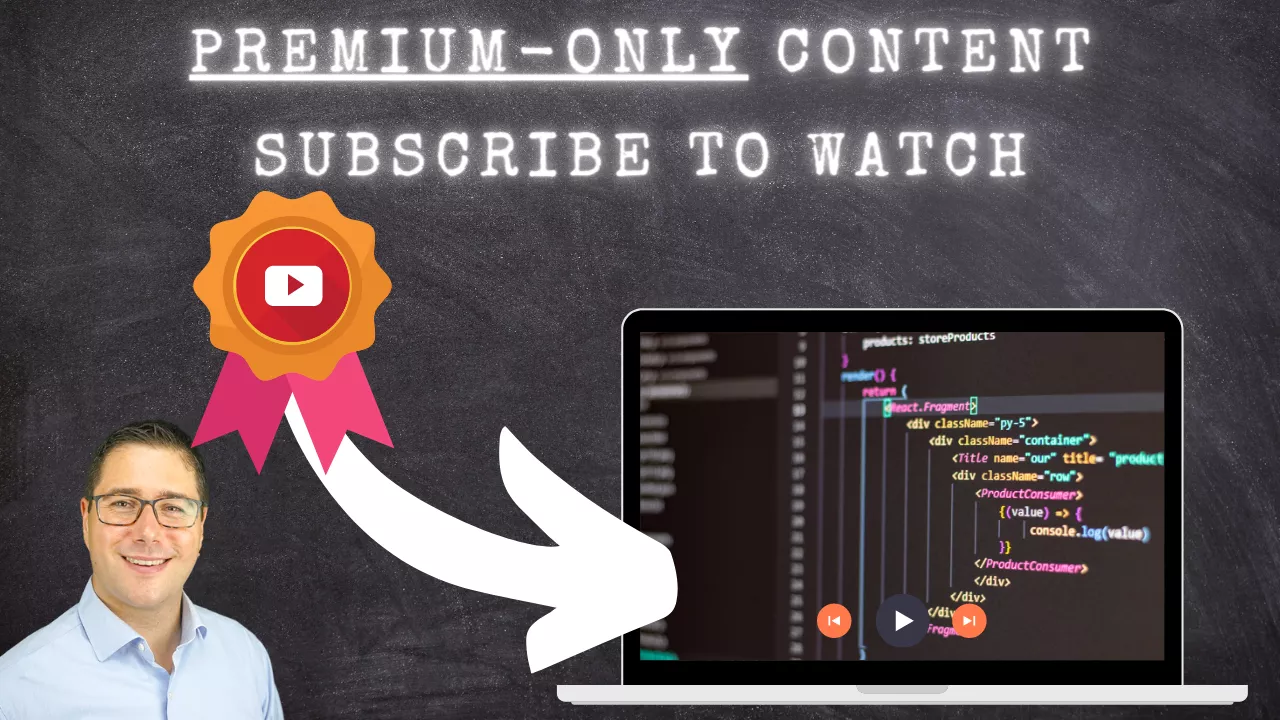
First, re-model the Frontend part:
render() {
if (!this.state.loaded) {
return <div>Loading Web3, accounts, and contract...</div>;
}
return (
<div className="App">
<h1>Capuccino Token for StarDucks</h1>
<h2>Enable your account</h2>
Address to allow: <input type="text" name="kycAddress" value={this.state.kycAddress} onChange={this.handleInputChange} />
<button type="button" onClick={this.handleKycSubmit}>Add Address to Whitelist</button>
</div>
);
}
Secondly, add the functions "handleInputChange" and "handleKycSubmit":
handleInputChange = (event) => {
const target = event.target;
const value = target.type === "checkbox" ? target.checked : target.value;
const name = target.name;
this.setState({
[name]: value
});
}
handleKycSubmit = async () => {
const {kycAddress} = this.state;
await this.kycContract.methods.setKycCompleted(kycAddress).send({from: this.accounts[0]});
alert("Account "+kycAddress+" is now whitelisted");
}
Also don't forget to change the state on the top of your App.js to modify the KYC Whitelist:
class App extends Component {
state = { loaded: false, kycAddress: "0x123" };
componentDidMount = async () => {
Finally, it should look like this:
The problem is now, your accounts to deploy the smart contract is in ganache, the account to interact with the dApp is in MetaMask. These are two different sets of private keys. We have two options:
-
Import the private key from Ganache into MetaMask (we did this before)
-
Use MetaMask Accounts to deploy the smart contract in Ganache (hence making the MetaMask account the "admin" account)
-
But first we need Ether in our MetaMask account. Therefore: First transfer Ether from Ganache-Accounts to MetaMask Accounts