Deployment with Truffle to Ganache¶
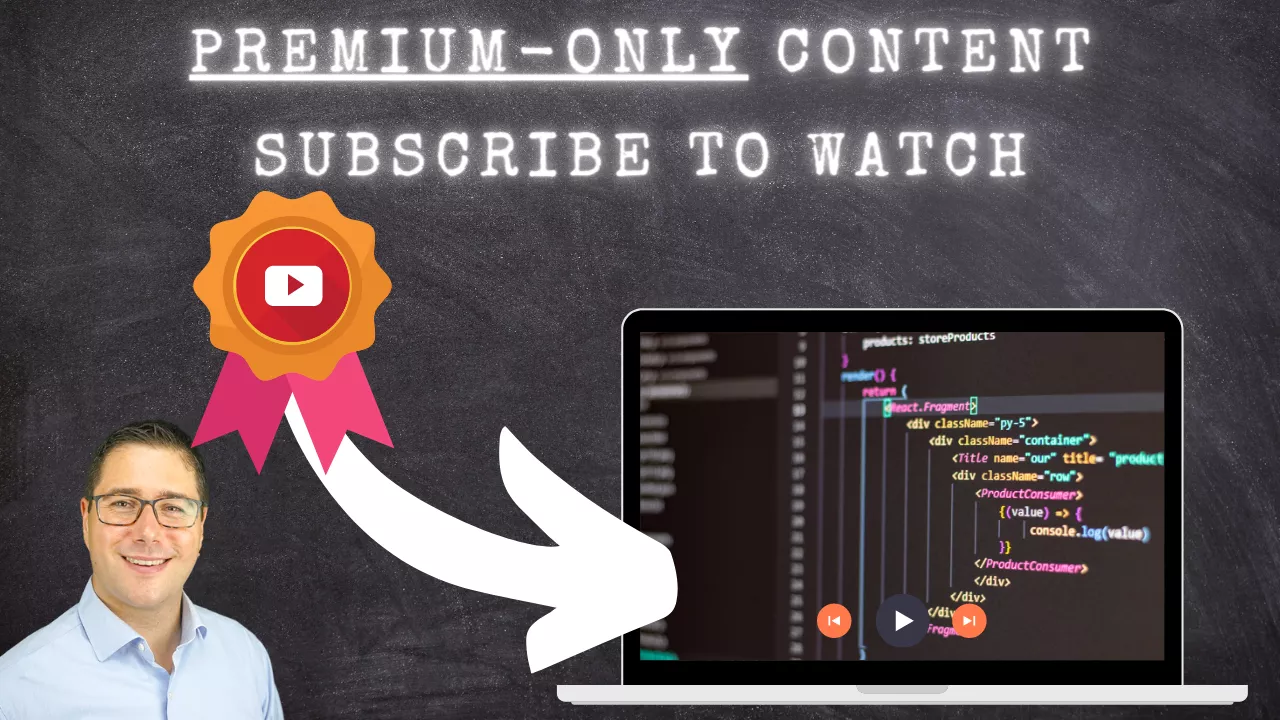
So, the initial idea with truffle was not to deploy smart contract, but to do migrations on the blockchain. Very much like database migrations, where you update a schema. That's why the migrations are scripts in the migrations
folder.
But that's not the only thing, because usually development work, unit testing and deployment (or migrations) go hand in hand.
The migrations file is a set of rules that lets truffle know how to deploy something.
In the truffle-config file, you define where to deploy it (which network) - or you use truffle dashboard, which we will do later.
The First Truffle Migrations File¶
Before going into Ganache and other networks, lets create a migrations file.
Create a new js file in the migrations folder called "01-spacebears-deployment.js" with the following content:
const Spacebears = artifacts.require("Spacebear");
module.exports = function(deployer) {
deployer.deploy(Spacebears);
}
What happens under the hood is that truffle will load each JavaScript file, ordered by the number prefix. The artifacts.require function will scan the contents of the build/contracts folder for json files and load the corresponding contracts so they can be used in the js file.
Then it will use the default export and call it and inject a deployer.
The deployer has a few functions, but before we explain that, let's try and deploy our token.
The Truffle Development Console¶
Truffle comes with a built in development console. It features a test-network with 10 accounts and 100 Eth each. It's very similar to the JavaScript VM from Remix, but also opens a port and an interactive JavaScript console where you can actually interact with smart contracts.
Start it on the terminal with
truffle develop
You will get an interactive console where you can type in commands.
To run the migrations on the development network here, completely forgoing the truffle-config.js
file you can simply type in
migrate
And truffle will run the migrations and deploy the Spacebears contract on the chain:
In this particular lab, I want to talk about deployment to Ganache. The development console is really cool, but the console in general only shines if we deploy the token to different networks.
Exit the Development Console with
.exit
You'll see next how that works with Ganache.
What is Ganache?¶
All three, Truffle, Hardhat and Foundry come with their own flavor of Development-Chains. All of them have a few features you'll love, but none of them has all features.
Let's start with Ganache, because that belongs to the Truffle ecosystem and is the oldest one.
Ganache comes in two flavors: A version with a Gui and an installer and a nodejs version that is installed via NPM.
They're function-wise equal and both go back to the project in the Github Repository.
Truffle vs. Hardhat Misconceptions
There's a lot of rant about truffle and ganache, especially from the Harthat community. This goes way back to the early days where Hardhat was just rebranded from Buidler and had addressed a few shortcomings from Truffle.
Hardhat was (and is) in a lot of aspects much faster than truffle, especially with unit-testing (more on that later).
One of the most praised features from Hardhat was the console.log
feature, where one could use this directly from within solidity and console log variables. This meant, no debugging needed and the workflow is pretty much the same as in any JavaScript app. Just put in a console.log wherever you want and see the variable content, no matter if the transaction fails or not.
That is now available in Ganache too.
The other problem was that truffle did have an on-chain migrations contract. That meant, for each deployed contract, truffle would write another transaction into the migrations contract to save the migration on chain. This is also deactivated by default.
Installing and starting Ganache¶
Ganache is installed actually quite easily. Especially on the command line:
npm install -g ganache
That's basically all there is. You can use Ganache in a lot of different ways, but I simply suggest to install it globally and treat it like an external program.
If you want to run ganache, you simply type in
ganache
and it should come up with 10 accounts. Very much like the truffle development network. The difference is Ganache has a lot more configuration options which we are using later on. Like mainchain forking etc...
Using Ganache¶
The big question is, how do you get your smart contracts now in Ganache?
That is, by instructing Truffle to use Ganache as one of the networks through the truffle-config.js
.
If you open the truffle-config.js file, you see a networks section where most is commented out.
And if you have a look in the console, you see that ganache-cli opens an RPC listener on Port 8545, which is also the standard port for Ethereum.
Let's add a network called "ganache" to the truffle-config.js file. Add this to the networks section
module.exports = {
networks: {
ganache: {
host: "127.0.0.1", // Localhost (default: none)
port: 8545, // Standard Ethereum port (default: none)
network_id: "*", // Any network (default: none)
},
}
}
Then open a second terminal, while ganache is still running and type in
truffle migrate --network ganache
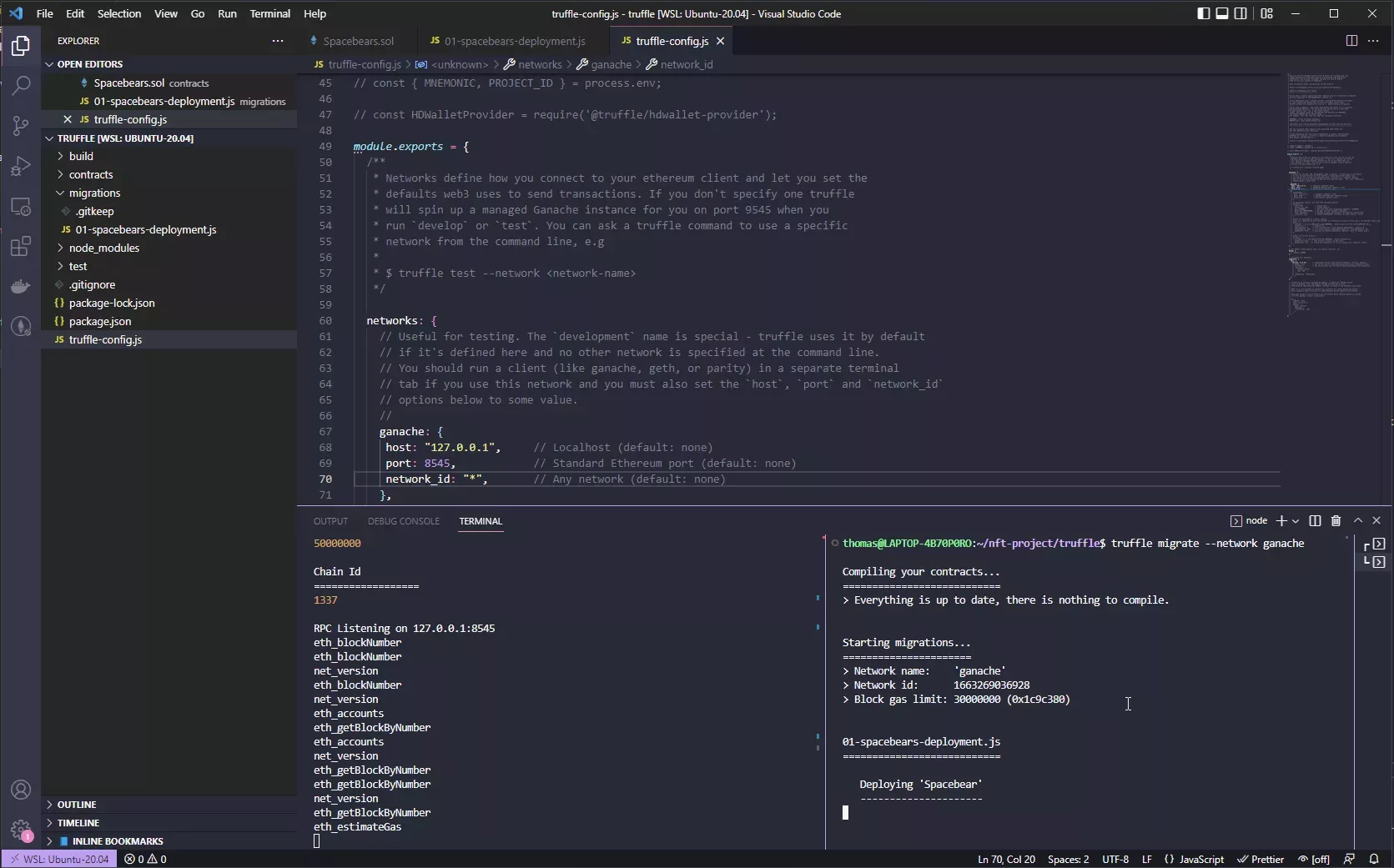
Okay, now your smart contract is on Ganache. But what now? How to interact with it?
Truffle has an interactive JavaScript console to do just that, which is what we're going to use in the next lab.