Understanding Web3.js - Introduction¶
It's time to go beyond Solidity a bit. In this section we're introducing events, and it makes no sense to learn them without seeing the benefits directly in web3js. So, in this lecture I want to give you a little introduction to Web3.js. Particularly I want to cover the following things here:
- Understanding the components involved in deploying a Smart Contract with Web3js
- How to interact with a Smart Contract using Web3.js
We're going to use Remix for this and build upon the already existing web3js deploy script.
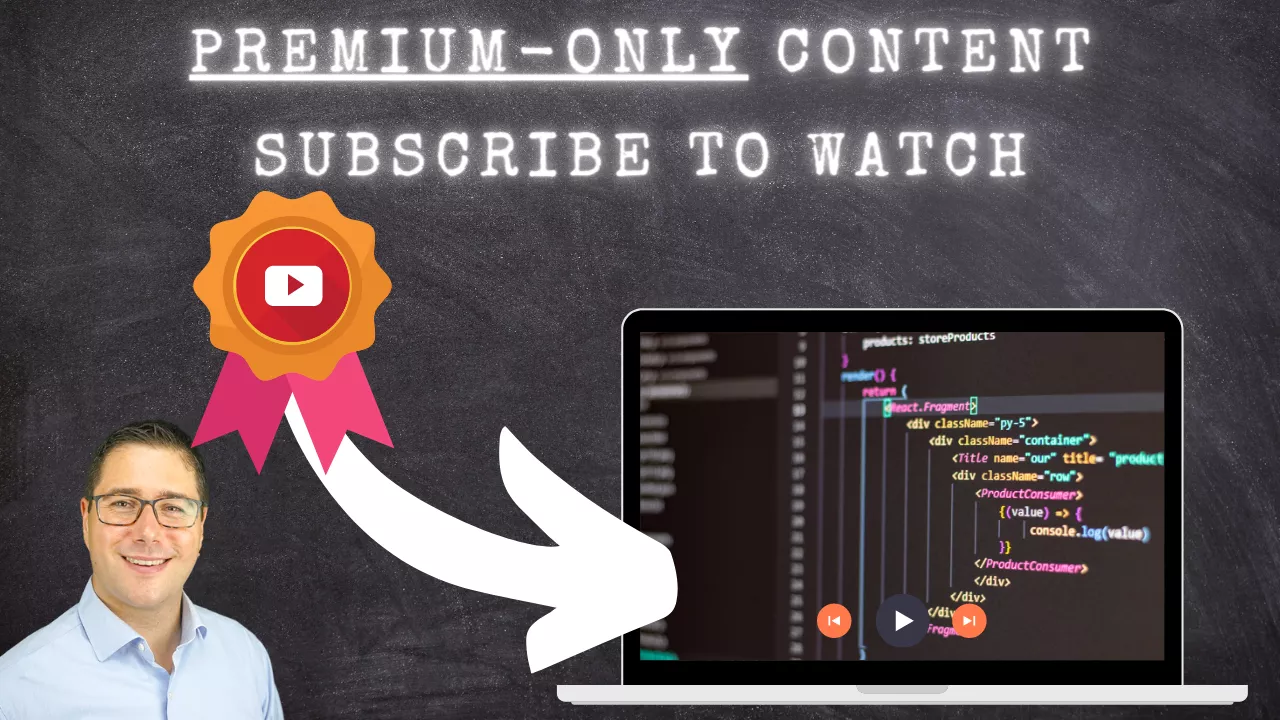
What is Web3.js?¶
Web3.js is a JavaScript library that lets you interact with a blockchain node via its RPC interface. That can be a RESTful HTTP interface, or WebSockets.
Web3.js is logically grouped into components, trying to somehow map the RPC interface definition. If we look at a typical blockchain node, go-ethereum, you will see certain namespaces https://geth.ethereum.org/docs/rpc/server. And if you direct your attention the Ethereum Page about JSON-RPC, you see that there are functions like eth_blockNumber
and eth_getBalance
to query (or even write) information from/to the blockchain.
If we have a closer look at Web3.js you see that there's a component called web3-eth, which you can reach then under web3.eth
. There are JavaScript functions to interact with a blockchain node, for example web3.eth.getBalance(...)
. By calling this JavaScript function, the web3 library would go talk to the Blockchain Node you're connected to and execute a JSON RPC call called eth_getBalance
with the parameter.
From the ethereum.org website, this would be an example using curl:
// Request
curl -X POST --data '{"jsonrpc":"2.0","method":"eth_getBalance","params":["0x407d73d8a49eeb85d32cf465507dd71d507100c1", "latest"],"id":1}'
// Result
{
"id":1,
"jsonrpc": "2.0",
"result": "0x0234c8a3397aab58" // 158972490234375000
}
Web3 Providers: HTTP vs. WebSocket vs. IPC¶
In the early days, WebSockets were not supported really well. Most connections were made with a standard HTTP polling method. Right now, the most standard way for a web-app is the WebSocket provider. WebSockets have the major advantage that there is no polling needed, you can subscribe to certain events as you will see in the next lecture.
Web3.js is not sending the requests directly, it abstracts it away into these providers. If you use MetaMask then one of the providers is already injected into the website itself. So, using this is, in the end, exactly the same as connecting directly to a blockchain node.
In Remix, via the command line (or terminal?!) you can directly interact with web3js.
web3.eth.getAccounts()
Will give you a list of accounts available. If you have selected the Remix VM in the environment-dropdown in the Deploy & Run Transaction tab, it will give you the accounts that are available throught he accounts-dropdown. If you have selected Injected Web3-Provider it will most likely give you the account that is in MetaMask.
Unfortunately the javascript console isn't very good for scripting. For example, in the current version of remix, this fails:
accounts = web3.eth.getAccounts();
console.log(accounts); // {}
accounts //[...]
accounts[0] //Unexpected token u in JSON at position 0
What works is, if you are actually scripting the commands in a javascript file.
Scripting in Remix¶
If you head over to the File Explorer, you can already see two scripts in the scripts-folder. Both demonstrate how you can deploy a smart contract using web3.js or ethers.js.
Let's add a new script in there to output the first account in the accounts-list.
Add a new file called get_accounts.js
with the following contents:
(async () => {
let accounts = await web3.eth.getAccounts();
console.log("Accounts: ", accounts);
console.log("Accounts 1:", accounts[0]);
})();
This runs an anonymous main function, which is using the async/await syntax to work with promises (web3.eth.getAccounts()
actually returns a promise which needs to be resolved). It then outputs all accounts and account #1 (which is at index 0). If you right click on the script and hit "run" it will run and the output is visible in the console area:
Global Objects
Wondering where "web3" comes from? It is one of many objects that are globally injected in Remix.
If you are working with React or Vue, you would need to download and install web3js yourself (npm install ... or yarn install...).