Understanding (Unsigned) Integers¶
In case you don't know what exactly integers are, then let's define first what we're talking about:
An integer is [...] a number that can be written without a fractional component. For example, 21, 4, 0, and −2048 are integers, while 9.75, ... is not. https://en.wikipedia.org/wiki/Integer
Integral types may be unsigned (capable of representing only non-negative integers) or signed (capable of representing negative integers as well) https://en.wikipedia.org/wiki/Integer_(computer_science)
In layman's terms: Integers are numbers without decimals. Unsiged integers cannot be negative.
Let's define a simple Smart Contract first so we can set an integer and then read the value again.
Smart Contract¶
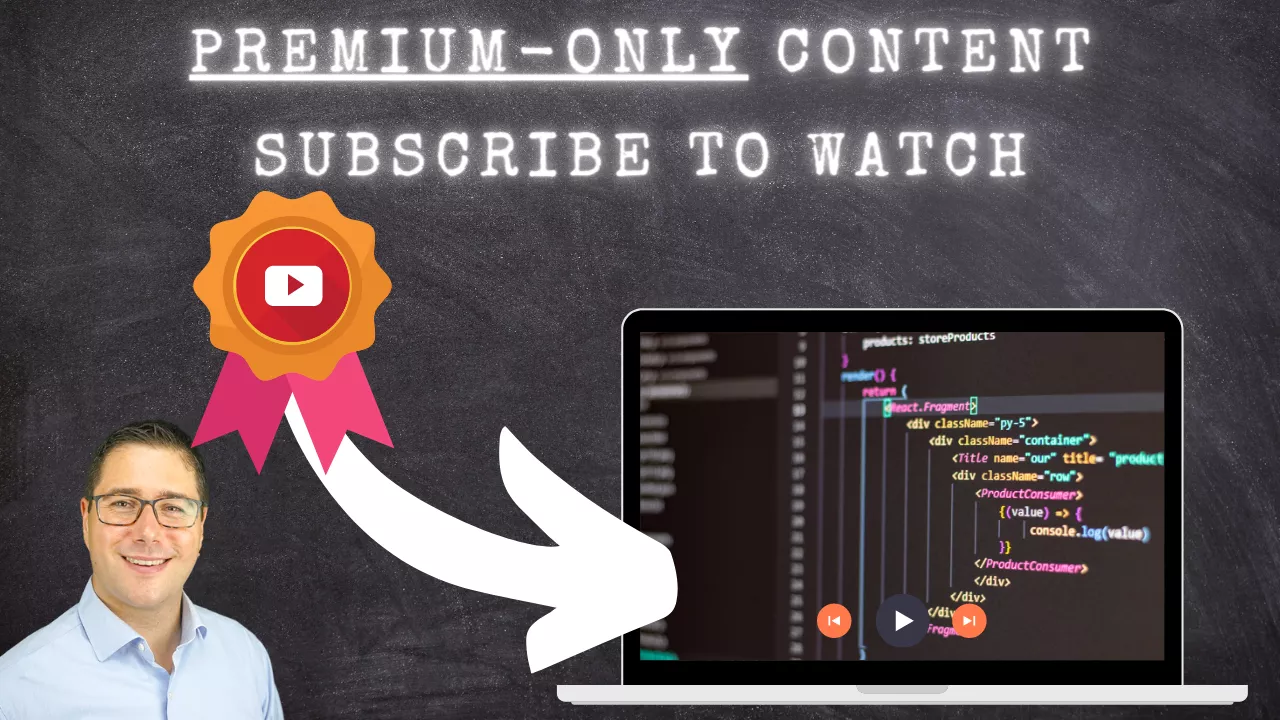
Create a new file and name it IntegerExample.sol and fill in the following content:
// SPDX-License-Identifier: GPL-3.0
pragma solidity ^0.8.1;
contract IntegerExample {
uint public myUint;
}
There are two important things to see here:
- The variable is not initialized, but as we will see in a moment, still has a default value
- A public variable automatically creates a getter function in solidity.
Deploy the Smart Contract¶
Open the "Deploy & Run Transactions" Plugin. Make sure the correct Smart Contract is selected from the dropdown. Then simply hit "Deploy":
You will be able to observe a few things:
-
A new transaction will be sent and you can see that in the console of Remix (bottom right).
-
Your Smart Contract is available in the "Deploy & Run Transactions" Plugin, at the bottom. You might need to uncollapse it.
You can now interact with your smart contract.
Interact with the Smart Contract¶
To interact with your Smart Contract, Remix is automatically generating buttons for every function. If you click on "myUint", you call the auto-generated getter fucntion from the public variable called "myUint".
Standard Workflow
This is a standard workflow, change the smart contract, redeploy. Currently, there are no in-place updates. For every change we do, we have to deploy a new version of the Smart Contract.
Write a Setter Function¶
// SPDX-License-Identifier: GPL-3.0
pragma solidity ^0.8.1;
contract IntegerExample {
uint public myUint;
function setMyUint(uint _myUint) public {
myUint = _myUint;
}
}
Deploy a new version of the Smart Contract - simply hit "Deploy" again. You wil see that you have two instances of your Smart Contract on the bottom of the "Deploy & Run Transactions" Plugin. You can safely close the old version - the new version is on the bottom.
If you click on "myUint" it will be initially 0 again.
Let's update it to 5. You can enter the number "5" into the input field next to "setMyUint", then click on "setMyUint":
If you do so, you can again observe the console of Remix on the bottom right that it sent a transaction.
When you click on "myUint" now, it should show you "5" instead of "0".
When you click on a function that only reads a value, then no transaction is sent to the network, but a call. You can see this in the console on the right side again.
Transaction vs Call
A transaction is necessary, if a value in a Smart Contract needs to be updated (writing to state). A call is done, if a value is read. Transactions cost Ether (gas), need to be mined and therefore take a while until the value is reflected, which you will see later. Calls are virtually free and instant.
Great! Now you know the basic workflow, how to deploy and how to update your code during development. But working only with Integers is a bit boring. Let's level up a bit and learn some more types before doing our first project.
Please note: In the next sections I will silently assume you are deploying a version of a Smart Contract and delete the old instance, whenever we do some changes. I recommend repeating this a few times so you don't forget it.