Deploying a Smart Contract with Foundry¶
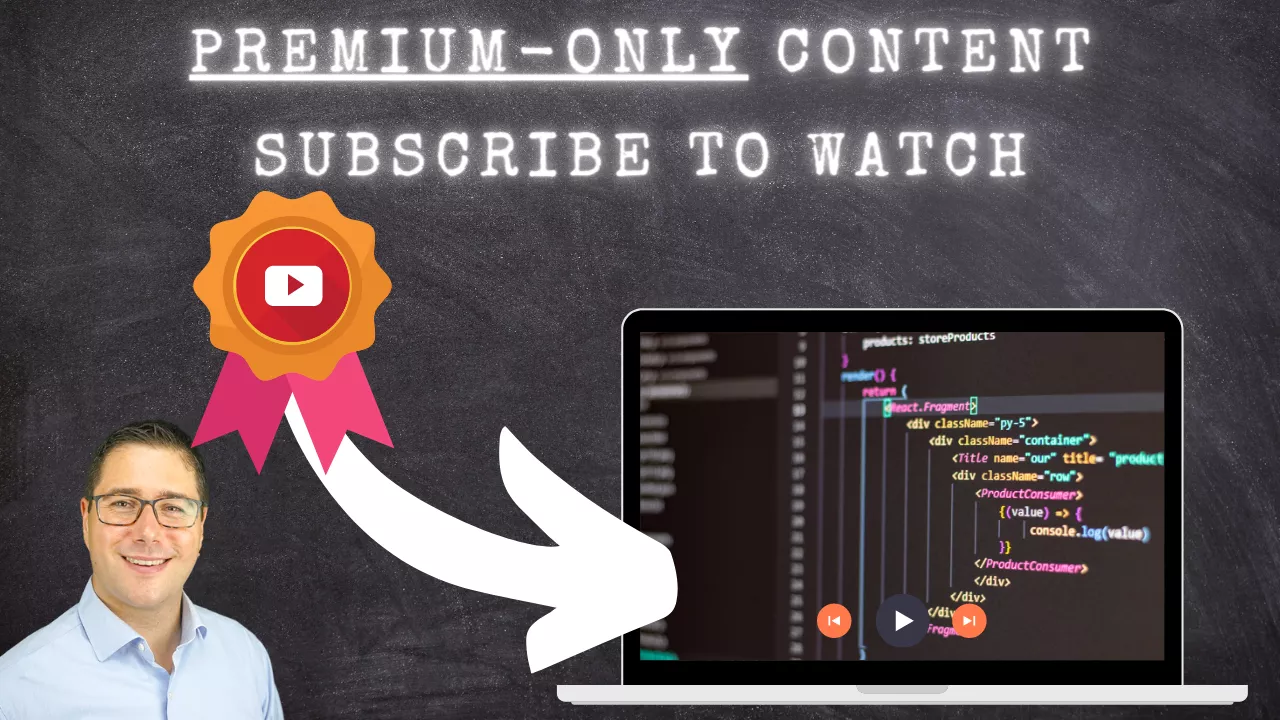
In this project we're going to deploy our Smart Contract using Foundry scripts.
Let's get started by adding a script to the script folder, called "Deploy.sol":
pragma solidity ^0.8.4;
import "forge-std/Script.sol";
import "../src/Spacebear.sol";
contract SpacebearScript is Script {
function setUp() public {}
function run() public {
string memory seedPhrase = vm.readFile(".secret");
uint256 privateKey = vm.deriveKey(seedPhrase, 0);
vm.startBroadcast(privateKey);
Spacebear spacebear = new Spacebear();
vm.stopBroadcast();
}
}
Here we read the contents of the .secret file. Copy it over from hardhat or truffle, which we created earlier.
Then also add two things to a new .env file:
GOERLI_RPC_URL=https://goerli.infura.io/v3/[INFURA_KEY]
ETHERSCAN_API_KEY=...
Enter there the infura key we created earlier and the etherscan api key, if you want foundry to directly verify the contracts with etherscan.
One last thing is missing to actually let foundry read the contents of the .secret
file, we have to give it permissions. Change the content of the foundry.toml
file and add fs_permissions:
[profile.default]
src = 'src'
out = 'out'
libs = ['lib']
fs_permissions = [{ access = "read", path = "./"}]
# See more config options https://github.com/foundry-rs/foundry/tree/master/config
[rpc_endpoints]
goerli = "${GOERLI_RPC_URL}"
Running a Foundry Script¶
It's time to run the script. Before we can do that, we have to make sure the environment variables are set in our environment.
Run
source .env
inside the foundry folder to set the environment variables correctly.
Then you can run the forge script command to run the deploy script:
forge script script/Deploy.sol:SpacebearScript --broadcast --verify --rpc-url ${GOERLI_RPC_URL}
What should happen is that forge runs your solidity script. In that script it tries to broadcast the transaction. It writes it back into the broadcast folder in a run-latest.json
file:
Verifying Smart Contracts with Foundry¶
With Foundry the Contract verification works automatically, so you actually shouldn't need to do anything. You can check that by going to https://goerli.etherscan.io (or the testnet address you used) and check the contract yourself.