Deploying Smart Contracts with Hardhat¶
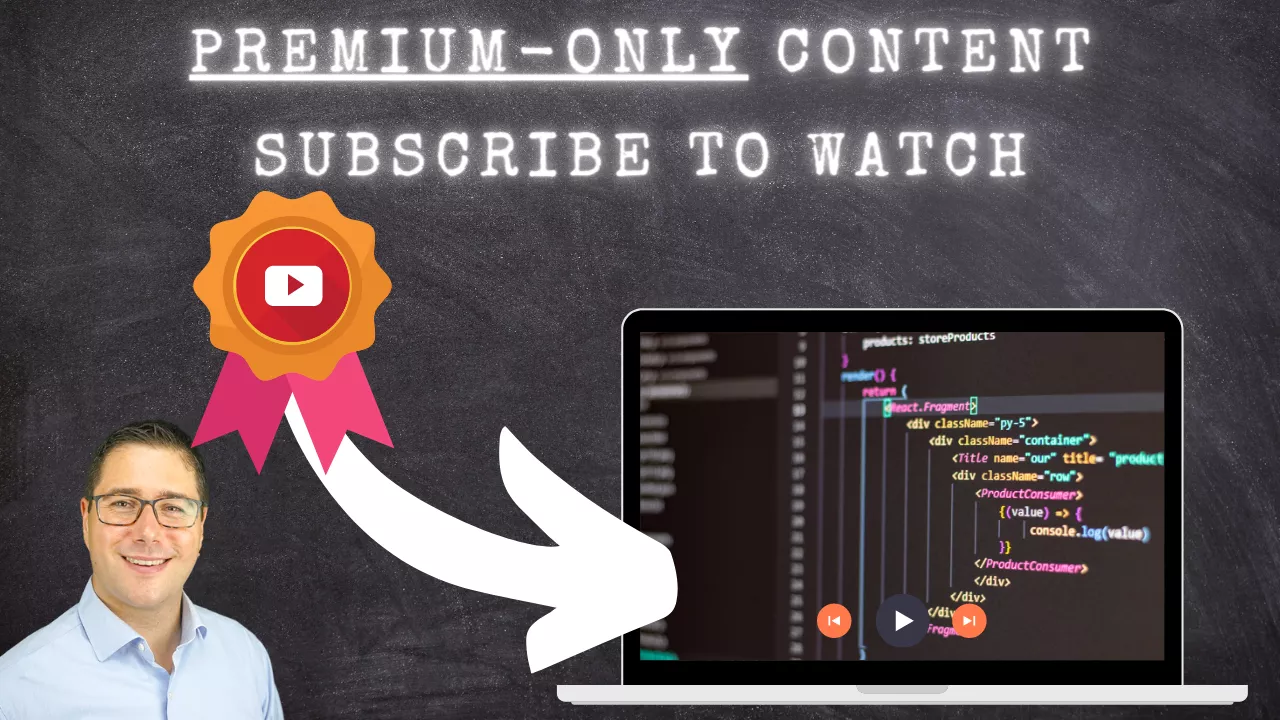
Now, let's see how we can deploy the Smart Contract on GΓΆrli (or any other network).
I hope you still have the Infura configuration ready, because that's what you need. And there's no Truffle-dashboard-shortcut, unfortunately.
Deploy Scripts Manually¶
With Hardhat, there are no migration scripts. At least not in the default out-of-box version. Everything is done via a normal scripts, which isn't specifically just for deployment. It can be really anything.
And Hardhat works, by default, with ethersjs. You can also use web3.js, but
Let's create the first script in scripts/deploy.js with the following content:
(async () => {
try {
const Spacebear = await hre.ethers.getContractFactory("Spacebear");
const spacebearInstance = await Spacebear.deploy();
await spacebearInstance.deployed();
console.log(
`Deployed contract at ${spacebearInstance.address}`
);
} catch (err) {
console.error(err);
process.exitCode = 1;
}
})();
Deployment with HardHat¶
Before we deploy to Goerli, lets deploy to the Ganache equivalent from Hardhat, the Hardhat local node:
Start a node in a terminal with
npx hardhat node
This opens a node in the terminal with port 8545 open:
Then we run the script on a second terminal to --network localhost:
npx hardhat run --network localhost scripts/deploy.js
Deployment to Goerli with HardHat¶
To deploy to Goerli, we need to add the network to the hardhat.config.js.
But in addition we need to supply our seed phrase and infura endpoint.
- copy the .infura and .secret files from your truffle project.
- Don't forget to add .infura and .secret to your .gitignore
- Update the hardhat.config.js to read the .infura and .secret files and deploy to goerli
GΓΆrli vs Sepolia
At the time of writing GΓΆrli might get deprecated in favor of Sepolia. GΓΆrli is an Proof of Authority network while Sepolia is the Proof of Stake clone from Mainnet Ethereum. Both networks have issues with their faucets. Best would be to use whatever network you can get Ether for.
require("@nomicfoundation/hardhat-toolbox");
const fs = require("fs");
let mnemonic = fs.readFileSync(".secret").toString().trim();
let infuraProjectID = fs.readFileSync(".infura").toString().trim();
/** @type import('hardhat/config').HardhatUserConfig */
module.exports = {
networks: {
goelri: {
url: "https://goerli.infura.io/v3/" + infuraProjectID,
accounts: {
mnemonic,
path: "m/44'/60'/0'/0",
initialIndex: 0,
count: 20,
},
},
},
solidity: "0.8.17",
};
Then simply run
npx hardhat run --network goerli scripts/deploy.js
Verifying the Smart Contract using HardHat¶
There is an integrated verification plugin in Hardhat. Let's quickly verify our smart contract.
- Add the .etherscan file to your hardhat project
- Add the .etherscan to .gitignore
- add the following parts to the hardhat.config.js
require("@nomicfoundation/hardhat-toolbox");
const fs = require("fs");
let mnemonic = fs.readFileSync(".secret").toString().trim();
let infuraProjectID = fs.readFileSync(".infura").toString().trim();
/** @type import('hardhat/config').HardhatUserConfig */
module.exports = {
networks: {
goerli: {
url: "https://goerli.infura.io/v3/" + infuraProjectID,
accounts: {
mnemonic,
path: "m/44'/60'/0'/0",
initialIndex: 0,
count: 20,
},
},
},
etherscan: {
apiKey: fs.readFileSync(".etherscan").toString().trim(),
},
solidity: "0.8.17",
};
Deploy using Hardhat-Deploy¶
This is, of course, just the basic method to deploy contracts so far. There are a plethora of addons to persistently store the contract address. A sample implementation is in the hardhat boilerpate to store "frontend files".
A more sophisticated version is the hardhat-deploy plugin, which you can see over here, but exceeds what we can do in this course for now: https://github.com/wighawag/hardhat-deploy/tree/master