Destroying Smart Contracts using selfdestruct¶
The data on the blockchain is forever, but the state is not. That means, we can not erase information from the Blockchain, but we can update the current state so that you can't interact with an address anymore going forward. Everyone can always go back in time and check what was the value on day X, but, once the function selfdestruct()
is called, you can't interact with a Smart Contract anymore.
Let's try this!
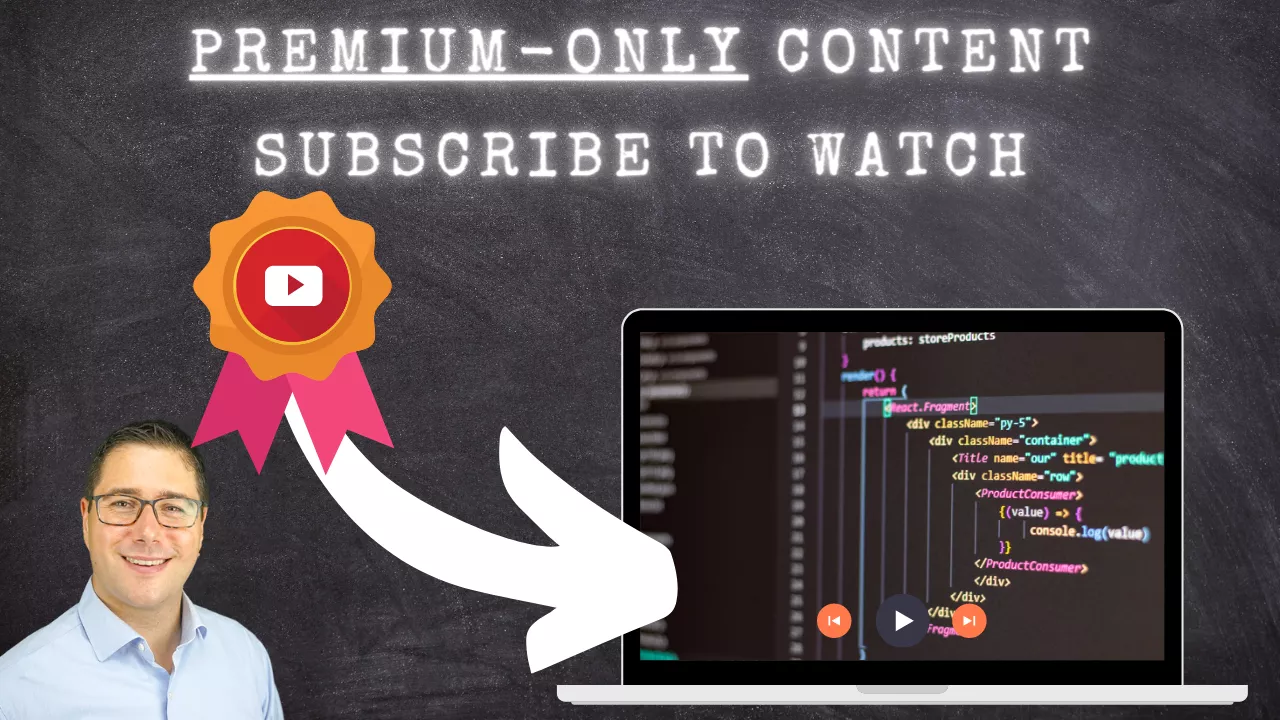
Potential Removal of SELFDESTRUCT
There might be an Ethereum Protocol update coming ahead which removes the SELFDESTRUCT functionality all-together. As writing this, it's not out there (yet), but might be soon, so take the following lab with this in mind.
Sample Smart Contract¶
Let's use this Smart Contract with a selfdestruct
function. The selfdestruct
function takes one argument, an address. When selfdestruct is called, all remaining funds on the address of the Smart Contract are transferred to that address. It's important to note, that the EVM will transfer funds to that address no matter what. So, even if another smart contract is the target address and that smart contract doesn't define a payable receive function, he will still receive the funds.
//SPDX-License-Identifier: MIT
pragma solidity 0.8.16;
contract StartStopUpdateExample {
receive() external payable {}
function destroySmartContract() public {
selfdestruct(payable(msg.sender));
}
}
How does selfdestruct
work? It's a function, that takes one argument, an address, which receives all funds stored on the contract address. Then it will remove the contract code from state. The address of the contract is then empty going forward.
The contract should be easily readable and the only surprise will be, what happes when you interact with the smart contract after it has been destroyed. Once you call destroySmartContract
, the address of the Smart Contract will contain no more code. You can still send transactions to the address and transfer Ether there, but there won't be any code that could send you the Ether back.
Let's try this!
Try our new Smart Contract¶
- Deploy a new Instance
- Send 1 Ether to the smart contract
- Try to call
destroySmartContract
and 1 Ether is sent back.
A Transaction should go through smoothly!
Interacting with Destroyed Smart Contracts¶
Now comes the part that is puzzling a lot of newcomers to Ethereum.
What happens if you try to send Ether again to the smart contract? Will there be an error or not?
There won't be an error! Internally you are sending Ether to an address. Nothing more.
Try it! Use the same contract instance, don't redeploy. Just send 1 Ether.
Works perfectly fine!
But try to get your Ether out again!
Also this transaction goes through smoothly! But wait!
Check your Balance!
It isn't updated...
You locked one Ether at the Smart Contract address for good. There's no more code running on that Address that could send you the Funds back.
So, be careful with the Contract life-cycle!
Re-Deployment of Smart Contracts to the Same Address
Once scenario, which is not in this particular course videos, is in-place upgrades. Since the CREATE2 Op-Code was introduced, you can pre-compute a contract address. I was talking about this extensively in the Proxy contract minicourse. If you are interested - head over to the mini-course section! Warning: it is quite advanced though...
Without CREATE2, a contract gets deployed to an address that is computed based on your address + your nonce. That way it was guaranteed that a Smart Contract cannot be re-deployed to the same address.
With the CREATE2 op-code you can instruct the EVM to place your Smart Contract on a specific address. Then you could call selfdestruct(), thus remove the source code. Then re-deploy a different Smart Contract to the same address.
This comes with several implications: when you see that a Smart Contract includes a selfdestruct()
then simply be careful. Those implications will become more and more apparent as you progress through the course, especially when we talk about the ERC20 Token allowance. At this stage it is too early to discuss them all, but if you want to read on about it, checkout this article.
Alright, that's enough of a detour into advanced topics. Let's continue with something more easy and straight forward: Source Code Verification