The Truffle JavaScript Console¶
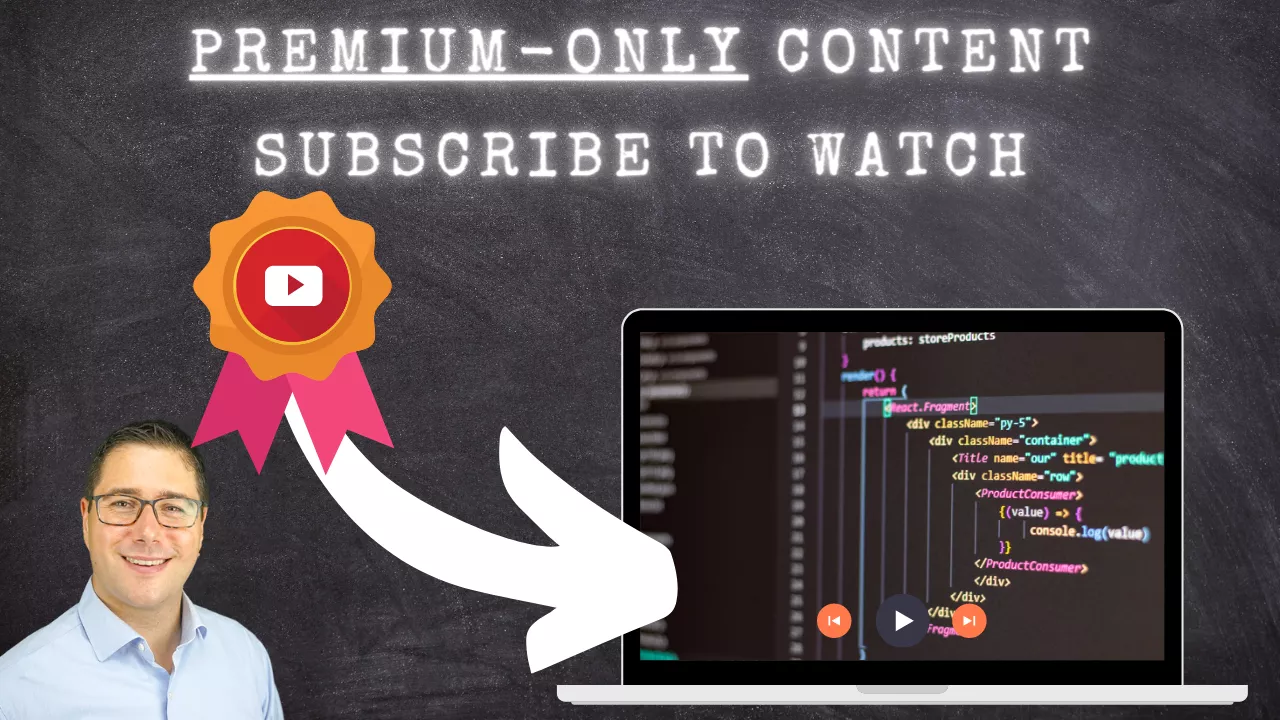
Truffle has an integrated and interactive JavaScript console. We're going to use that now to
- connect to a blockchain node
- mint an NFT
- view the NFT details
Connecting to a Blockchain Node¶
In the previous lecture we deployed the smart contracts by running truffle migrate --network ganache
.
The console is very similar to start:
truffle console --network ganache
It will open an interactive JavaScript console:
Then you are connected to the network.
Inside the console, you can access web3js and for example query the available accounts:
web3.eth.getAccounts();
Minting an NFT using the Truffle Console¶
Here's what a lot of people completely confuses: You can interact with your smart contracts using the truffle console. But you don't use web3 contracts - it uses truffle-contracts. I don't know how long it stays like this, but historically, when web3 was still in version 0.20, truffle added truffle-contracts, an overlay over web3js. It allowed for easy interaction with smart contracts and fixed a few shortcomings of web3 0.20.
Nowadays web3 1.x or the even newer versions are all using promises etc. The biggest difference between truffle-contract and web3js is probably how you access functions and events.
In web3js you access functions with contractInstance.methods.functionName()
and in truffle-contract you access that with contractInstance.functionName()
.
Let's run an example, a lot of magic happening under the hood.
Create a new contract instance:
const spacebear = await Spacebear.deployed()
If you run
spacebear.name()
It will make a call (which you can see in Ganache) and return the name stored in the contract:
This way you can also mint an NFT. Let's mint an NFT for account#2 with account#1:
const accounts = await web3.eth.getAccounts();
await spacebear.safeMint(accounts[1], "spacebear_1.json");
You see, you don't even have to tell truffle-contract which account you want to use to send the transaction, because it automatically assumes you take the first account to send the transaction.
View NFT Details¶
Now that you have access to the NFT, its easy to view the NFT details.
spacebear.ownerOf(0);
spacebear.tokenURI(0);
This way we could also test specific aspects of a token. For example, we could check that the token URI matches what we would expect, or that the owner is set correctly.
Of course, for an already tested token contract from openzeppelin it makes little sense (although approaching 100% test coverage is always good), but for other projects - for example the morpher ecosystem or any other custom contracts that merely utilize the blockchain - are more than just a nice-to-have.
Let's look in the next lab how we can write unit-tests for truffle.