Integers and Unsigned Integers¶
What are Integers?¶
In case you don't know what exactly integers are, then let's define first what we're talking about:
An integer is [...] a number that can be written without a fractional component. For example, 21, 4, 0, and −2048 are integers, while 9.75, ... is not. https://en.wikipedia.org/wiki/Integer
Integral types may be unsigned (capable of representing only non-negative integers) or signed (capable of representing negative integers as well) https://en.wikipedia.org/wiki/Integer_(computer_science)
In layman's terms: Integers are numbers without decimals. Unsiged integers cannot be negative.
Integer and Usigned Integer Ranges in Solidity¶
In Solidity there are several integers sizes available, which mostly influence the amount of gas used to store a value.
The smallest one is (u)int8 (0 to 2^8-1, or 0 to 255), the biggest one is uint256 (0 to 2^256 - 1, or 0 to a "very large number" 🤔)
Signed Integer Range
Unsigned Integers in Solidity going from 0 to 2^256-1. However, signed integers need one bit to store the sign of the number (negative or positive). This is why they are not just shifted from the uint range 0-2^256-1 to -2^128 to +2^128-1, but to -2 ** 255 to 2 ** 255 - 1.
Let's define a simpleSmart Contract first so we can set an integer and then read the value again.
Video¶
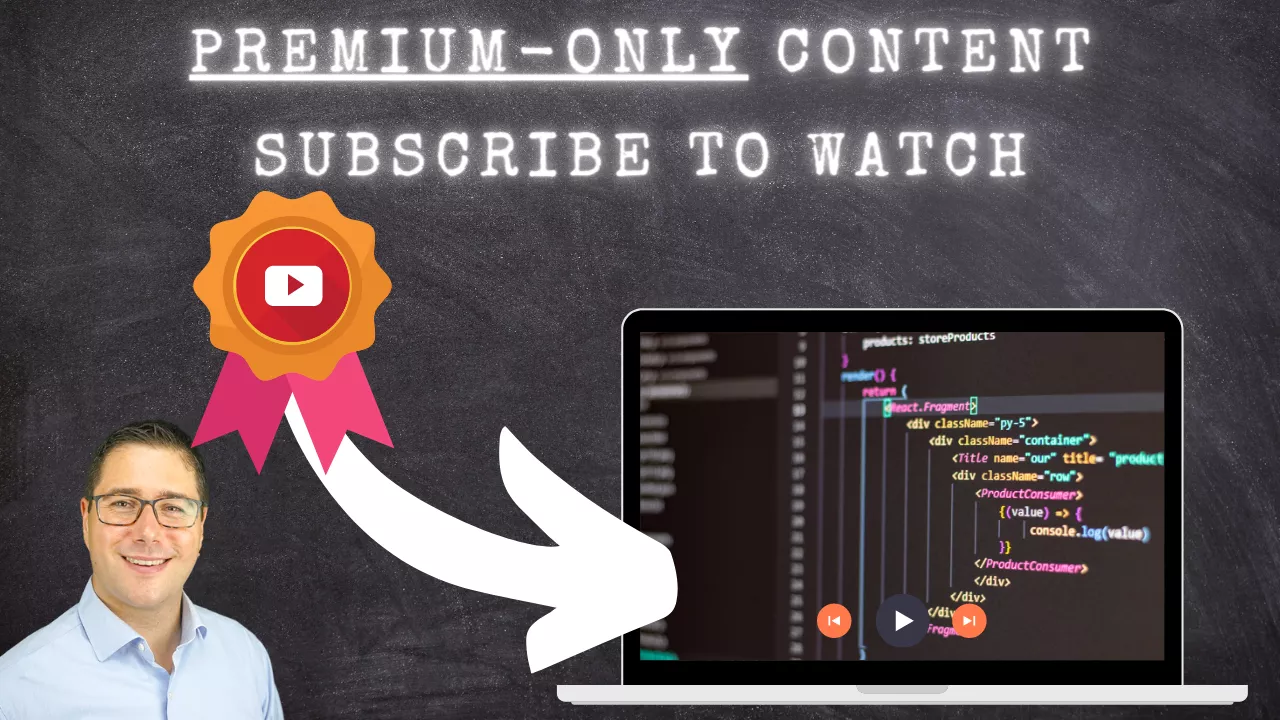
Sample Code¶
//SPDX-License-Identifier: MIT
pragma solidity 0.8.14;
contract ExampleUint {
uint256 public myUint; //0 - (2^256)-1
uint8 public myUint8 = 250;
uint8 public myUint8_EXP = 2**4; //=16, exponentiation is done with **
int public myInt = -10; //-2^255 to 2^255 - 1
function setMyUint(uint _myUint) public {
myUint = _myUint;
}
function decrementUint() public {
myUint--;
}
function incrementUint8() public {
myUint8++;
}
function incrementInt() public {
myInt++;
}
}
Run through the sample code! Make sure you understand the difference between uint8 and uint256. Next, make sure you understand that in Solidity versions >0.8.x an (unsigned) integer cannot roll over.
One more thing I didn't mention in the video:
Rounding with Integers
Integers in Solidity are always rounded down! If we divide e.g. 100 / 101 = 0.990... but in Solidity the result will be 0. Integers in Solidity are actually cut off (not even rounded), in other words always rounded down.
That's all there is. Play around with integers and then head over to the next lecture where we talk about integer rollovers more in-depth!